The easiest way to integrate Youtube Iframe API in Angular & React
Youtube iframe API is tricky to integrate when it comes to Component architecture. Here is an easy way to integrate Youtube Iframe API in Angular & React
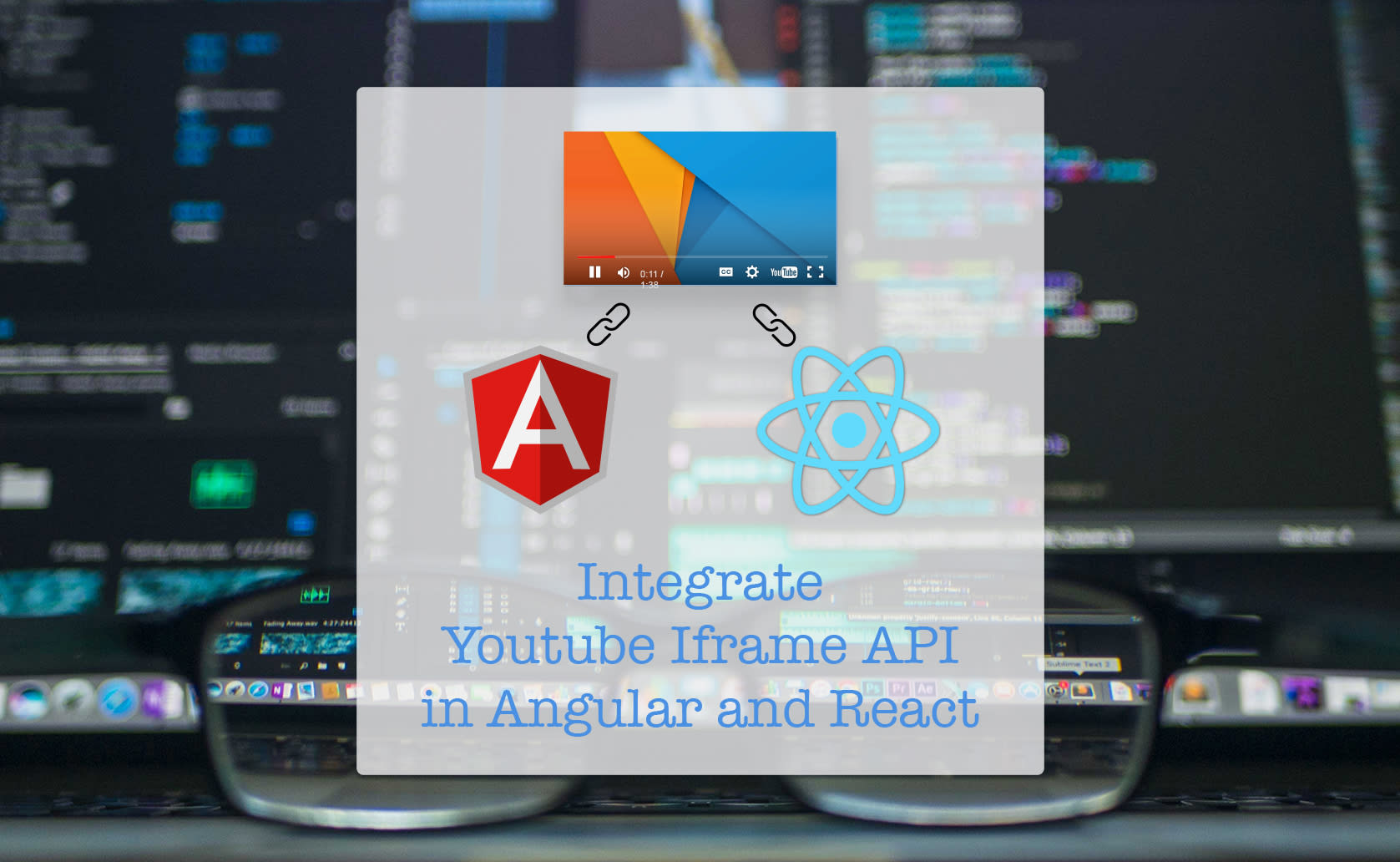
Youtube iframe API is tricky to integrate when it is to be done with Component-based architecture.
Here is the easiest way to integrate Youtube Iframe API in Angular and React
And the code samples below include the use of reframe.js
/noframe
.
reframe.js
and noframe
are the from https://github.com/dollarshaveclub/reframe.js to make the no-responsive iframe content responsive.
Angular
Demo
Code
import { Component, OnInit } from '@angular/core';
import reframe from 'reframe.js';
@Component({
selector: 'app-track',
template: `<div class="max-width-1024">
<div class="embed-responsive embed-responsive-16by9" id="player">
</div>
</div>`,
styles: [`.max-width-1024 { max-width: 1024px; margin: 0 auto; }`],
})
export class TrackComponent implements OnInit {
public YT: any;
public video: any;
public player: any;
public reframed: Boolean = false;
constructor() { }
init() {
var tag = document.createElement('script');
tag.src = 'https://www.youtube.com/iframe_api';
var firstScriptTag = document.getElementsByTagName('script')[0];
firstScriptTag.parentNode.insertBefore(tag, firstScriptTag);
}
ngOnInit() {
this.init();
this.video = '1cH2cerUpMQ' //video id
window['onYouTubeIframeAPIReady'] = (e) => {
this.YT = window['YT'];
this.reframed = false;
this.player = new window['YT'].Player('player', {
videoId: this.video,
events: {
'onStateChange': this.onPlayerStateChange.bind(this),
'onError': this.onPlayerError.bind(this),
'onReady': (e) => {
if (!this.reframed) {
this.reframed = true;
reframe(e.target.a);
}
}
}
});
};
}
onPlayerStateChange(event) {
console.log(event)
switch (event.data) {
case window['YT'].PlayerState.PLAYING:
if (this.cleanTime() == 0) {
console.log('started ' + this.cleanTime());
} else {
console.log('playing ' + this.cleanTime())
};
break;
case window['YT'].PlayerState.PAUSED:
if (this.player.getDuration() - this.player.getCurrentTime() != 0) {
console.log('paused' + ' @ ' + this.cleanTime());
};
break;
case window['YT'].PlayerState.ENDED:
console.log('ended ');
break;
};
};
//utility
cleanTime() {
return Math.round(this.player.getCurrentTime())
};
onPlayerError(event) {
switch (event.data) {
case 2:
console.log('' + this.video)
break;
case 100:
break;
case 101 || 150:
break;
};
};
}
React
Demo
Code
class MyAppComponent extends React.Component {
constructor(props) {
super(props);
this.init();
this.video = '1cH2cerUpMQ' //video id
window['onYouTubeIframeAPIReady'] = (e) => {
this.YT = window['YT'];
this.reframed = false;
this.player = new window['YT'].Player('player', {
videoId: this.video,
events: {
'onStateChange': this.onPlayerStateChange.bind(this),
'onError': this.onPlayerError.bind(this),
'onReady': (e) => {
if (!this.reframed) {
this.reframed = true;
reframe(e.target.a);
}
}
}
});
};
}
render() {
const style = `.max-width-1024 { max-width: 1024px; margin: 0 auto; }`;
return (<div>
<style>{style}</style>
<div className="max-width-1024">
<div className="embed-responsive embed-responsive-16by9" id="player">
</div>
</div>
</div>
);
}
init() {
var tag = document.createElement('script');
tag.src = 'https://www.youtube.com/iframe_api';
var firstScriptTag = document.getElementsByTagName('script')[0];
firstScriptTag.parentNode.insertBefore(tag, firstScriptTag);
}
onPlayerStateChange(event) {
console.log(event)
switch (event.data) {
case window['YT'].PlayerState.PLAYING:
if (this.cleanTime() == 0) {
console.log('started ' + this.cleanTime());
} else {
console.log('playing ' + this.cleanTime())
};
break;
case window['YT'].PlayerState.PAUSED:
if (this.player.getDuration() - this.player.getCurrentTime() != 0) {
console.log('paused' + ' @ ' + this.cleanTime());
};
break;
case window['YT'].PlayerState.ENDED:
console.log('ended ');
break;
};
};
//utility
cleanTime() {
return Math.round(this.player.getCurrentTime())
};
onPlayerError(event) {
switch (event.data) {
case 2:
console.log('' + this.video)
break;
case 100:
break;
case 101 || 150:
break;
};
};
}
ReactDOM.render(<MyAppComponent />, document.getElementById('app'));
Happy Coding.
Conclusion
Let me know through comments ? or on Twitter at @heypankaj_ and/or @time2hack
If you find this article helpful, please share it with others ?
Subscribe to the blog to receive new posts right in your inbox.
This post is sponsored by PluralSight
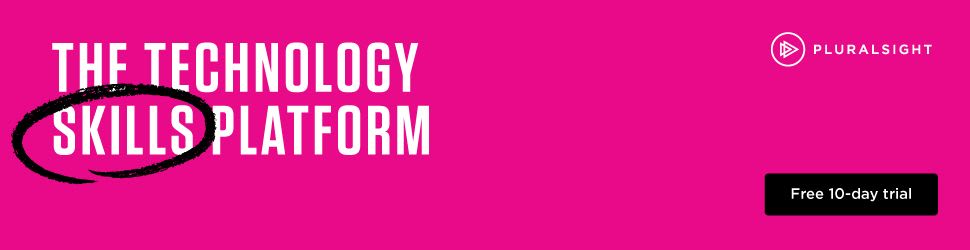