Browser Storage and AngularJS
Storage is an important part of an application. Let's see how to use Browser Storage & AngularJS with localStorage and sessionStorage.
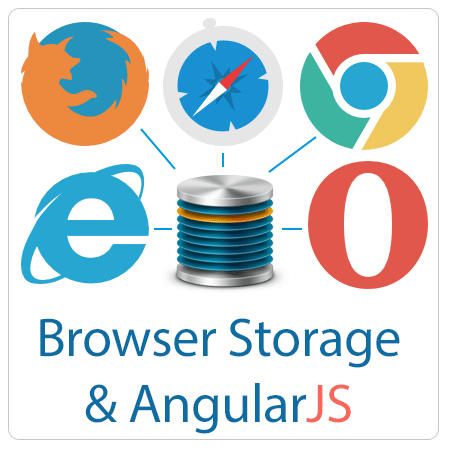
Storage is an essential part of an application. And as the web is now an application platform, storage options should be known. So we are going to discuss Browser Storage and AngularJS implementation of Browser Storage. So the client-side storage; we will be dealing with; are sessionStorage and localStorage. And to handle these properly in AngularJS, we will also go through Factories and Services in AngularJS.
Session Storage (sessionStorage
)
The session storage is used to store the information available for the page session duration. A page session lasts as long as the browser is open and survives over page reloads and restores. Opening a page in a new tab or window will cause a new session to be initiated. And it is available to use as window.sessionStorage
. To check whether you can use them sessionStorage
or not; you can use window.Storage
.
Local Storage (localStorage
)
The localStorage
is same as sessionStorage
but it is persistent, and information will sustain after the page is closed. localStorage
is available to use as window.localStorage
and its availability can be checked in a similar way to sessionStorage
i.e. by using window.Storage
localStorage and sessionStorage can be seen in Chromeโs Web Inspector in the Resources tab, as shown below:
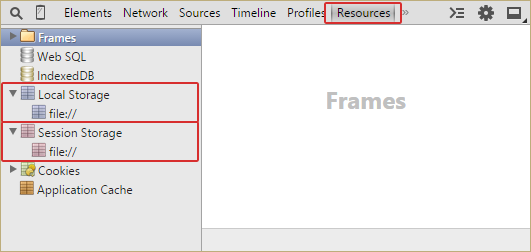
AngularJS way
In AngularJS, nothing is available as a direct object to any controller or any handler function. As AngularJS had a dependency injection mechanism, everything has to be passed as a dependency. So to use Storage in our web app, we need to use it through the window
object. And to use window
object; we need to pass it as a dependency to out code blocks like controllers/factories as $window
.
And it will be very clumsy to handle data directly with $window
passed as a controller dependency. So we will take the help of providers.
AngularJS Providers and Factories
Providers are the data interfaces in angular. You can create providers to interface your application from any source of data. The source can be any remote location, REST API, device hardware etc. Providers can be of various kinds like services, factories, values etc.
We will create factory providers to access data from the Storage. These factories, which will create an easy interface for controllers to access data from local/session storage, will have access to the dependencies to access data from and return an object. This object may contain other functions to facilitate sub-methods like get, set etc. Here is the basic code for creating a factory in AngularJS.
Now let's see how the Storage works in the browser. So initially, we will check its availability, i.e. local or session storage is available or not. For that, we can check by window.Storage
.
if(window.Storage){
/* handle storage */
}
Now, if itโs available, we can create the key-value pairs in the storage. To create, we can use setKey
, and to read the value, we can use getKey
function. All the critical functions to work with Storage are listed below:
- setItem: This function accepts two parameters; the first one is the name of the key and the second one is the value that needs to be stored against the key; thus function returns the status.
- getItem: This function accepts one parameter, which is the name of the key, and it will return the string value stored against that key. If it fails to get the value, then it will return false.
- removeItem: This function accepts one parameter, which is the name of the key, and it will remove that key. If it fails to get the value, then it will return false.
- clear: This function accepts no parameter and will remove all the items in the storage.
For Example:
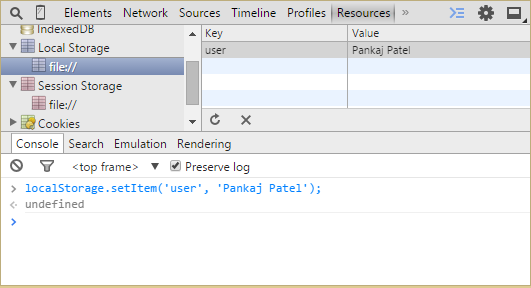
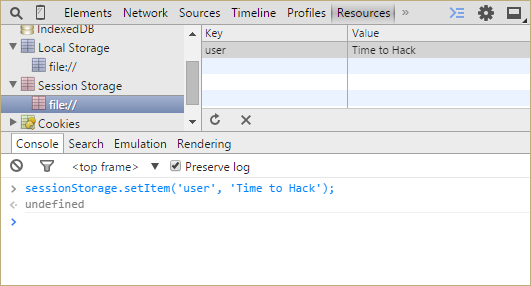
So that was a basic code to work with the local and session storage in the browser. Let's go ahead to handle these with the angularJs factories, but before that, here is a minimal example of the factory in angularJs:
And the complete storage handling code for both sessionStorage and localStorage is as follows:
Now let's see the above angular storage module in action. we will use this storageModule
as a dependency in the ToDo app we created in the previous post. And then, to store and get the todo task; we will use the storage module; the code looks as follows:
You can see the working demo of this app by going to the demo link, and you can download the files:
Conclusion
In the above code, we needed only session storage and only one key to be stored and updated, so we refactored the factory code according to the todo app needs.
Let me know through comments ? or on Twitter at @heypankaj_ and @time2hack
If you find this article helpful, please share it with others ?
Subscribe to the blog to receive new posts right in your inbox.